Functions and Methods in Python
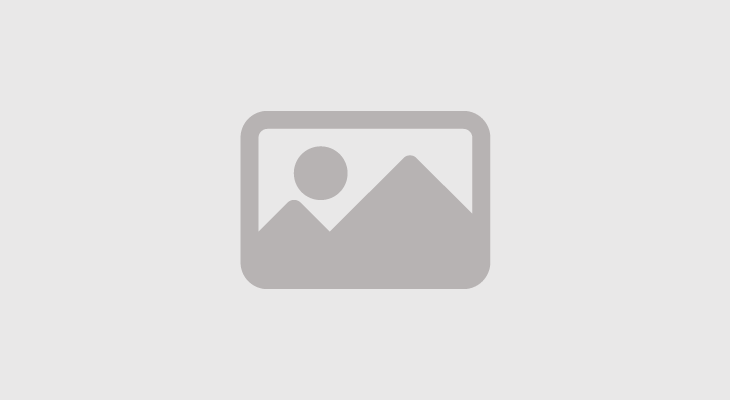
Welcome to the realm of functions and methods in Python! Functions and methods are essential constructs in programming that encapsulate a set of instructions to perform a specific task. In Python, these constructs provide modularity, code reusability, and a clear organizational structure, contributing to the overall readability and maintainability of your code.
Functions in Python
A function in Python is a named block of code that performs a specific task. It can take input parameters, execute a sequence of statements, and optionally return a result. Functions are integral to decomposing complex problems into manageable, reusable components, promoting a modular and efficient coding style.
Methods in Python
Methods, closely related to functions, are functions that are associated with an object. In Python, objects have methods that can be invoked to perform actions or retrieve information specific to that object. For example, strings have methods for string manipulation, and lists have methods for list operations.
Modularity and Code Reusability
Functions and methods contribute to the modularity of code by allowing you to break down a program into smaller, self-contained units. This modular approach facilitates code reuse, making it easier to maintain and extend your programs without duplicating code.
Parameter Passing
In Python, parameters can be passed to functions and methods, allowing for flexibility and customization. Functions can be designed to accept various inputs, and return values can provide output based on the specified parameters.
Built-in and User-Defined Functions
Python provides a rich set of built-in functions, such as print()
, len()
, and max()
, which are readily available for common tasks. Additionally, you can define your own functions to encapsulate custom logic tailored to the specific requirements of your programs.
Encapsulation and Abstraction
Functions and methods enable encapsulation, allowing you to hide the internal details of a process and expose only the essential functionalities. This abstraction enhances code readability and comprehension, making it easier to collaborate on projects and maintain codebases.
As you delve into the world of functions and methods in Python, you'll discover their pivotal role in structuring your code, promoting reusability, and facilitating effective problem-solving. Whether you're working with built-in functions or creating your own custom functions and methods, Python's approach to encapsulation and modularity empowers you to write clear, concise, and maintainable code. Enjoy exploring the capabilities and elegance that functions and methods bring to the Python programming experience.
Functions and Methods in Python
Functions and Methods in Python are a fundamental and vital part of the programming world using this language. Functions and methods are powerful tools that help developers organize and efficiently reuse code. In simple terms, functions represent sets of programming commands that can be defined and called to perform specific tasks. Functions are defined using the keyword "def," followed by the function's name and a list of parameters if applicable, and then a colon.
As for methods, they are a special type of function associated with objects in Python. Methods are called on these objects to perform specific actions or operations related to these objects. Methods allow interaction with objects and performing related tasks.
Regardless of your experience levels in programming with Python, functions and methods play a crucial role in improving code organization and increasing reusability. If you are a beginner, these concepts will be the starting point to understand how to structure programs and organize commands. If you are an experienced programmer, a solid understanding of these tools will enhance your ability to build more powerful and complex applications by effectively leveraging functions and methods.
The concept of Functions and Methods in Python
Functions and methods are fundamental and vital components in the world of programming using the Python language. Understanding the concept of functions and methods is of utmost importance for any developer learning Python. Let's examine these concepts in detail:
Functions
- In Python, functions are sets of programming commands that allow developers to organize their code and reuse it efficiently. Functions are defined using the "def" keyword, followed by the function name and a list of parameters (if any), and then a colon.
- Functions represent independent executable units that perform specific tasks. For example, a function can be defined to sum a set of numbers or to print a specific text on the screen.
- When a function is called in the code, the commands inside it are executed, and a value is returned if the "return" statement is defined within the function. Functions can be called at multiple points in the code to perform the same task repeatedly.
Methods:
- Methods are a special type of functions in Python that are associated with specific objects. Here, the object is an instance of a class, and methods are the functions that can be called on these objects.
- For example, if we have an object of the "Car" class, this object can have many methods such as "start," "stop," and "change_speed." These methods can be called on the object to perform specific actions.
- Methods allow for organizing code and breaking it down into small units that deal with specific operations related to the object. They increase code reuse, simplify maintenance, and improve the program's structure.
In summary, functions and methods in Python are powerful means of organizing and reusing code, as well as improving the understanding and efficiency of the program. Whether you are a beginner or a professional programmer, you will find that using functions and methods is essential for developing robust and organized Python applications.
Differences Between Functions and Methods in Python
1. Definition and Usage
- Functions: Functions are defined using the "def" keyword, followed by the function name and a list of parameters if needed. They can be called from anywhere in the code to perform a specific task. When a function finishes executing, it can return a value if the "return" statement is defined within the function.
- Methods: Methods are functions that are associated with specific objects and are used to perform actions related to those objects. To define methods, the "def" keyword is used within a class.
2. Invocation
- Functions: Functions can be directly called by using the function name and passing the necessary arguments. For example: `result = add_numbers(5, 3)`, where "add_numbers" is the function name.
- Methods: Methods are called on objects using the dot notation with the object name and method name. For example: `car.start()`, where "start" is the method name and "car" is the object.
3. Relationship with Objects
- Functions: Functions are not tied to specific objects and can be called independently of any object
- Methods: Methods are associated with specific objects and operate on or modify data stored within those objects. Methods represent the behavior of the object.
4. Parameters
- Functions: Parameters can be passed to functions for use within the function and manipulation.
- Methods: Methods typically have a "self" parameter as the first parameter, which allows them to access data and variables stored within the object.
5. Location in Code
- Functions: Functions can be defined anywhere in the code and called from anywhere else.
- Methods: Methods are defined within classes and are part of the object's structure. They are only called on objects created from those classes.
How to Define Functions in Python
Defining functions in Python is a fundamental part of the programming process, as functions are used to encapsulate a set of code commands to perform a specific task. Functions are defined using the "def" keyword, followed by the function name, an optional list of parameters, and a colon. Here are the steps to define functions in Python:
1. Use the "def" keyword:
Start the function definition by writing the "def" keyword at the beginning.
2. Name the function:
After "def," specify the function's name. Choose a meaningful name for the function that reflects its main purpose.
3. Parameter list (inputs):
If the function needs to use information from outside, you can specify a list of parameters enclosed in parentheses. These parameters represent inputs that can be passed to the function when calling it.
4. Colon:
After naming the function and defining parameters (if any), use a colon to indicate the beginning of the code block that will be executed inside the function.
5. Write code inside the function:
Write the programming commands you want to execute inside the function after the colon. This code may include algorithms and operations that will be performed on the parameters if they are passed.
6. Use the "return" statement (optional):
If the function is expected to return a specific result, you can use the "return" statement with a value to return. If "return" is used, the function will terminate and return the specified value.
7. Call the function:
Once you have defined the function, you can call it from anywhere in your code by writing the function name and passing any required arguments. For example: `result = my_function(parameter1, parameter2)`
Once you've defined a function, you can use it to repeatedly execute commands without having to rewrite them each time. Functions are a powerful tool for organizing and reusing code, making programming more efficient.
Calling Functions and Reusing Them
It is an important part of Python programming. Once you have defined a function, you can use it in multiple places in your program without the need to rewrite the code repeatedly. Here's how to call functions and reuse them:
1. Calling a Function:
To use a specific function, you must call it by using the function's name and providing the required arguments if any.
For example, if you have a function that adds two numbers:
def add_numbers(a, b):
result = a + b
return result
You can call this function like this:
sum_result = add_numbers(5, 3)
In this example, the `add_numbers` function is called with arguments 5 and 3, and the result is stored in the variable `sum_result`.
2. Reusing Functions:
Reusing functions means using the same function in multiple places in your program without having to rewrite the code. This makes code organization and efficiency easier.
For example, if you have a function to calculate the average grade:
def calculate_average(grades):
total = sum(grades)
average = total / len(grades)
return average
You can call this function in different parts of your program to calculate different averages without rewriting the code:
math_scores = [85, 90, 92, 88, 78]
science_scores = [95, 88, 89, 92, 87]
math_average = calculate_average(math_scores)
science_average = calculate_average(science_scores)
In this example, the `calculate_average` function is called twice with different sets of grades to calculate the averages.
Calling functions and reusing them make programming more efficient and organized, allowing you to use the code you've written once in multiple places without repetition, reducing errors, and aiding in better program management.
Parameters in Functions: Input and Output
Parameters in functions are the values that you can pass to the function when calling it. They are used to pass data to the function to perform the required operations on that data. Here are more details on parameters in functions:
1. Input:
- Parameters are the values that you can pass to the function when calling it. These parameters can be of any data type, such as integers, strings, lists, etc.
- Parameters help customize the behavior of the function as needed. For example, if you have a function that adds numbers, you pass the numbers you want to add as parameters.
- You can define multiple parameters in one function and use them inside the function to perform different operations.
2. Output:
Functions often produce a result or an output value that reflects the work they do. Functions can return specific values.
- The `return` keyword in Python is used to return a value from the function. When the function reaches a `return` statement, it stops executing and returns the specified value.
- The returned value can be used at the calling site to utilize the result or store it in a variable for later use.
For example, if you have a function to sum a list of numbers:
def sum_numbers(numbers):
total = sum(numbers)
return total
In this example, the `sum_numbers` function takes a list of numbers as a parameter. When the function is called and a list of numbers is passed as a parameter, the function performs operations on this list and returns the total sum.
Understanding parameters in functions allows you to tailor or customize the behavior of functions so that you can perform the required operations with the appropriate data.
Built-in Methods in Python
Built-in methods in Python are a set of functions that come pre-packaged with the Python language and are available for use without the need to define them yourself. These methods provide useful and common functionalities that can be used in most programs. Here are some examples of built-in methods and their functions:
1. `print()`: This function is used to print text and information to the screen. You can pass the text you want to print as an argument to the `print()` function.
2. `len()`: It is used to count the number of elements in data structures such as lists, tuples, and strings.
3. `input()`: This function is used to obtain user input. The value entered by the user can be used in the program to make decisions or perform different operations.
4. `type()`: It is used to determine the type of a variable or value. When you pass a variable as an argument to this function, it returns the data type of that variable.
5. `range()`: This function is used to create a sequence of numbers. It can be used in loops to iterate over a specified range of numbers.
6. `max()` and `min()`: These functions are used to find the maximum and minimum values in a set of numbers.
7. `sum()`: It is used to calculate the sum of numbers in a list or tuple.
8. `str()`, `int()`, and `float()`: These functions are used to convert data from one type to another. For example, you can use `str()` to convert a number to a string.
9. `sorted()`: It is used to sort elements in a list or tuple based on their values.
10. `abs()`: This function is used to find the absolute value of a number. For example, `abs(-5)` returns 5.
11. `enumerate()`: It is used to get an index and value for each item in a list. It is useful when you need to track the position of items during iteration.
12. `zip()`: This function is used to combine elements from multiple lists into a single list.
13. `any()` and `all()`: These functions are used to check the truthiness of a condition among elements in a list. `any()` returns True if any element satisfies the condition, while `all()` returns True if all elements satisfy the condition.
14. `format()`: It is used to format strings and insert variable values into the text.
15. `open()`: This function is used to open and manipulate files.
These are just some examples of built-in functions in Python. You can use these methods to perform various tasks in your programs with ease and efficiency, and of course, you can also define your own functions to meet your specific needs.
How to Define Methods in Objects
Methods are an essential part of object-oriented programming in Python, allowing you to define and use functions associated with specific objects. Objects are like real-world entities with properties and behaviors. Here are steps to define methods in objects:
1. Define the Object: First, you need to define the object itself. This is done by creating a class using the `class` keyword. For example, if you want to create an object representing cars:
class Car:
pass
This code defines a class called `Car`, but it doesn't have any properties or methods yet.
2. Define Methods: Once you have defined the object, you can start defining the methods you want to include in the class. Methods should be defined within the class and use the `def` keyword. For example, if you want to add a method to start the car:
class Car:
def start(self):
print("The car is starting.")
In this example, a method named `start()` is defined within the `Car` class.
Calling Methods on Objects
Calling methods on objects in Python allows you to execute behaviors and actions associated with those objects. Here's how to call methods on objects without the need to write any code:
1. Create an Object: First and foremost, you need to create an object from the class that contains the methods you want to call. This is done by using the class name with parentheses, like so:
my_car = Car()
In this example, an object of the `Car` class is created and stored in the variable `my_car`.
2. Call the Method: After creating the object, you can call methods on the object using the dot notation (`.`), by writing the object's name followed by the method you want to call and parentheses. For example, if you have a method called `start()` in the `Car` class:
my_car.start()
This will invoke the `start()` method on the `my_car` object.
3. Method Parameters (Input): Sometimes, methods may require parameters to execute the intended behavior. When calling the method, you should pass the required parameters inside the parentheses. For instance, if you have a method that takes two parameters:
my_car.drive(50, "north")
In this example, the values 50 and "north" are passed as parameters to the `drive()` method.
4. Output (Return Value): Methods can also return values after execution. Objects can use these returned values after calling the method. For example, if you have a method that calculates distance and returns it:
distance = my_car.calculate_distance(2, 30)
In this case, the `calculate_distance()` method is called, and the returned value is stored in the `distance` variable.
These are the basic steps for calling methods on objects in Python. Calling methods allows you to execute behaviors and custom actions related to objects, making interactions with them efficient and effective.
Passing the Object Being Called on
In Python, you can directly pass the object being called on to the method itself as an argument, typically referred to as the `self` keyword. `self` is used to refer to the object itself within the method. Here are more details:
Method Definition: When defining a method inside a class, the first parameter (usually named `self`) should be the object on which the method will be called.
Method Invocation: When calling the method, you don't need to manually pass `self`. Python automatically does this when you call the method.
Using `self` Inside the Method: Inside the method itself, you can use `self` to access the variables and methods of the current object.
In conclusion, functions and methods in Python are essential and vital components in the world of programming using this language. Functions and methods are powerful tools that help developers organize and reuse code effectively, making programs more understandable, maintainable, and extensible.
Functions represent sets of programmable commands that can be defined and called to perform specific tasks, while methods are functions associated with objects in Python.
Summary
In summary, functions and methods are fundamental constructs in Python that facilitate modularity, code organization, and reusability. Functions are named blocks of code designed to perform specific tasks, accepting parameters and optionally returning values. Methods, closely related, are functions associated with objects, providing actions or information specific to those objects.
The modular nature of functions allows for the decomposition of complex problems into manageable units, promoting clearer code structure. This modularity enhances code reusability, enabling developers to efficiently build on existing functionalities without duplicating code.
Parameter passing in functions allows for flexibility and customization, enabling the creation of versatile and adaptable code. Python offers both built-in functions, readily available for common tasks, and the ability to define user-defined functions, tailoring code to specific project requirements.
Functions and methods contribute to encapsulation, hiding internal details and exposing only essential functionalities. This abstraction enhances code readability, simplifying collaboration and maintenance of codebases.
Whether working with built-in functions or creating custom functions and methods, Python's approach to encapsulation and modularity empowers developers to write clear, concise, and maintainable code. Enjoy harnessing the power of functions and methods for effective problem-solving and code organization in your Python programming endeavors.
Comment / Reply From
You May Also Like
منشورات شائعة
Newsletter
Subscribe to our mailing list to get the new updates!