Lists and Collections in Python
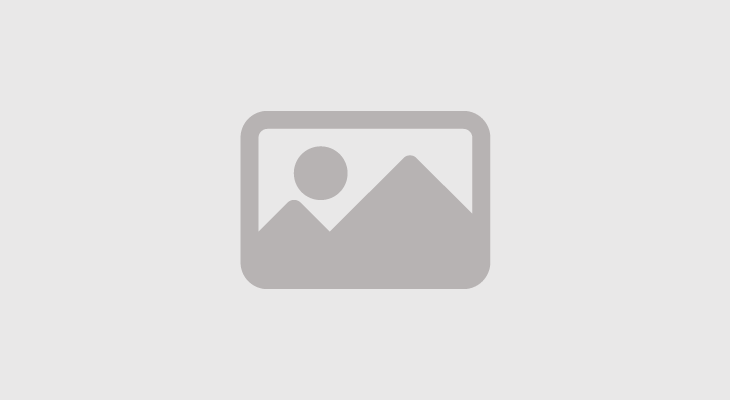
Welcome to the world of lists and collections in Python! Lists are versatile and dynamic data structures that allow you to organize and manipulate data in a flexible manner. In Python, lists are just one example of a broader category of collections, which include tuples, sets, and dictionaries, each serving distinct purposes in data organization and storage.
Lists in Python:
A list in Python is an ordered collection of items. It can hold elements of different data types and allows for easy indexing, slicing, and modification of its contents. Lists provide a fundamental way to manage and iterate through sequences of data, making them integral to various programming tasks.
Collections in Python
Beyond lists, Python offers several other collection types:
-
Tuples: Similar to lists but immutable, tuples are suitable for storing fixed sets of data.
-
Sets: Sets are unordered collections of unique elements, useful for mathematical operations like union, intersection, and difference.
-
Dictionaries: Dictionaries are key-value pairs that provide efficient data retrieval based on unique keys, offering a powerful way to represent structured information.
Dynamic Nature of Collections
One of the strengths of Python collections is their dynamic nature. Lists and other collection types can grow or shrink as needed, allowing for adaptability in handling data of varying sizes and structures.
Versatility in Data Handling
Collections in Python enable you to work with diverse types of data, from simple integers and strings to more complex structures. This versatility makes Python collections powerful tools for organizing, processing, and manipulating information in various domains.
Built-in Functions
Python provides a rich set of built-in functions and methods for working with collections. Whether it's sorting a list, checking membership in a set, or accessing values in a dictionary, these functions enhance the ease and efficiency of working with collections.
As you navigate through the world of lists and collections in Python, you'll discover their pivotal role in data manipulation and organization. Whether handling sequences of values, creating mappings, or managing unique sets of data, Python's collection types offer a flexible and efficient means to address a wide array of programming challenges. Enjoy exploring the capabilities and versatility that lists and collections bring to the Python programming experience.
Lists and Collections in Python
Python is a versatile programming language that provides various data structures for organizing and managing collections of data. Among these data structures, two of the most fundamental and widely used are lists and dictionaries, which are collectively referred to as collections.
Lists
Lists in Python: are ordered collections of items. They are created using square brackets `[]` and are capable of storing elements of different data types, including numbers, strings, and even other objects. Lists are versatile and play a crucial role in many Python programs. Here are some key characteristics and features of lists:
1. Ordered Sequence:
Lists maintain the order of elements as they are added. This means you can access elements by their position (index) in the list.
2. Mutable:
Lists are mutable, which means you can modify them after creation. You can add, remove, or change elements within a list.
3. Heterogeneous Elements:
Lists can contain a mix of different data types. For example, you can have integers, strings, and even other lists as elements of a single list.
4. Accessing Elements:
You can access elements of a list using their index. Python uses zero-based indexing, so the first element is at index 0, the second at index 1, and so on.
5. Slicing:
Lists support slicing, which allows you to create sublists by specifying a range of indices.
6. Common Operations:
Lists support various operations like appending, extending, inserting, and removing elements.
7. Length:
You can determine the number of elements in a list using the `len()` function.
Here's an example of creating and working with a list:
fruits = ['apple', 'banana', 'cherry']
print(fruits[0]) # Output: 'apple'
fruits.append('orange')
print(len(fruits)) # Output: 4
Dictionaries
While lists are useful for ordered collections, **dictionaries** are designed for key-value pairs. Dictionaries are created using curly braces `{}` or with the built-in `dict()` function. Here are some key characteristics and features of dictionaries:
1. Key-Value Pairs:
Dictionaries store data as key-value pairs, where each key is unique, and it maps to a corresponding value.
2. Unordered:
Unlike lists, dictionaries are unordered collections, meaning they do not maintain any specific order of elements.
3. Fast Access:
Dictionaries provide fast access to values based on their keys. You can look up a value by specifying its key.
4. Mutable:
Dictionaries are mutable, allowing you to add, modify, or remove key-value pairs.
5. Keys and Values:
Both keys and values in a dictionary can be of various data types. Keys are typically strings or numbers, but they can be any immutable type.
6. Common Operations:
Dictionaries support operations like adding new key-value pairs, modifying existing ones, and deleting entries.
Here's an example of creating and working with a dictionary:
student = {
'name': 'Alice',
'age': 25,
'courses': ['Math', 'Science']
}
print(student['name']) # Output: 'Alice'
student['age'] = 26
student['grade'] = 'A'
In summary, lists and dictionaries are fundamental collection data structures in Python, each serving different purposes. Lists are ordered and versatile for storing sequences of items, while dictionaries are used for key-value mappings, providing efficient access to data. Understanding how to use these collections is essential for writing Python programs effectively.
Concept of Lists in Python and How to Create Them
Lists in Python:
Lists are fundamental data structures in the Python programming language. They are used to store a collection of items or values in a specific order. You can think of a list as a container that holds multiple elements, where you can place any type of data, including numbers, text, and even other lists.
How to Create Lists:
1. Using Square Brackets [ ]:
To create a list in Python, you can use square brackets. For example:
my_list = [1, 2, 3, 4, 5]
In this example, a list is created containing five elements.
2. Accessing Elements:
You can access elements within a list by their index or position. In Python, indexing starts at 0, meaning the first element in the list can be accessed using index 0.
first_element = my_list[0] The value here will be 1
3. Storing Multiple Data Types:
You can store any data type inside a list, including numbers, text, variables, and even other lists. This makes lists very flexible data structures.
mixed_list = [1, "Hello", 3.14, True]
4. Lists are Mutable:
You can easily change the content of a list after creating it. You can add new elements, remove existing ones, and update the values of elements.
my_list.append(6) To add 6 to the list
my_list.remove(3) To remove the number 3 from the list
my_list[1] = "World" To update the second element to "World"
5. Working with Lists:
You can use loops and built-in functions to work with lists efficiently. For example, you can use a `for` loop to iterate through the elements of the list and perform various tasks.
for item in my_list:
print(item)
In summary, lists are essential data structures in Python used for organizing and storing data flexibly. You can create lists containing various data types and perform multiple operations on them, making them a powerful tool in your Python programming.
Adding and Removing Elements from Lists in Python
Adding Elements to Lists:
1. Using `append()`: You can add a new element to the end of a list using the built-in `append()` function, which takes the value you want to add as an argument.
my_list = [1, 2, 3]
my_list.append(4)
Now, the number 4 will be added to the list, and the list will look like this: `[1, 2, 3, 4]`.
2. Using `insert()`: If you need to add an element at a specific position within the list, you can use the `insert()` function. You pass the index (location) where you want to add the element as the first argument and the value you want to add as the second argument.
my_list = [1, 2, 3]
my_list.insert(1, 4)
In this example, the number 4 will be added at the second position in the list, and the list will become `[1, 4, 2, 3]`.
Removing Elements from Lists
1. Using `remove()`: To remove a specific element from the list based on its value, you can use the `remove()` function and pass the value you want to remove as an argument.
my_list = [1, 2, 3, 4]
my_list.remove(2)
Now, the number 2 will be removed from the list, and the list will become `[1, 3, 4]`. If there are multiple occurrences of the value you want to remove, only the first occurrence will be removed.
2. Using `pop()`: To remove and retrieve an element from the end of the list, you can use the `pop()` function without passing any arguments. The list will automatically remove the last element and return it to you.
my_list = [1, 2, 3, 4]
popped_item = my_list.pop()
Now, the number 4 will be removed from the end of the list, and the variable `popped_item` will contain the removed value.
3. Using `del`: You can use the `del` keyword to delete elements from the list based on their index. For example:
my_list = [1, 2, 3, 4]
del my_list[1]
This operation will delete the number 2 from the list, and the list will become `[1, 3, 4]`. You can also use `del` to delete the entire list.
These are some common ways to add and remove elements from lists in Python, and these operations provide great flexibility for managing data in your programs.
Utilizing Loops to Work with Lists in Python
Loops are a fundamental construct in programming that allows you to repetitively execute a series of operations or commands until a certain condition is met. When working with lists in Python, you can use loops to access the elements of the list and perform various operations on them. Here's how you can leverage loops to work with lists in Python:
1. `for` Loop for Accessing Elements:
The `for` loop is one of the most commonly used loops for working with lists. It allows you to iterate through all the elements of the list and perform actions on each element individually.
Example:
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
In this example, a `for` loop is used to iterate through the elements of `my_list` and print each item.
2. Using Index (Indexing):
You can also use indexing to access elements in the list. The `for` loop is used with `range()` to access the index, and using the index, you can access the corresponding values in the list.
Example:
my_list = [1, 2, 3, 4, 5]
for i in range(len(my_list)):
print(my_list[i])
Here, a `for` loop with `range()` is used to access the index, and then the index is used to access elements in the list.
3. `while` Loop:
The `while` loop is used when you need to execute operations based on a certain condition. It can be used to work with lists in a similar way to the `for` loop, but with a logical condition.
Example:
my_list = [1, 2, 3, 4, 5]
i = 0
while i < len(my_list):
print(my_list[i])
i += 1
Here, a `while` loop is used to access elements in the list based on a specific condition.
4. Performing Multiple Operations:
You can use loops to perform multiple operations on lists, such as searching for a specific item or applying a function to each element. For example, you can use a `for` loop to sum a set of numbers in the list.
Example:
my_list = [1, 2, 3, 4, 5]
total = 0
for item in my_list:
total += item
print(total)
In this example, a `for` loop is used to sum all the numbers in the list.
With loops, you can efficiently and effectively organize and manipulate data in lists in Python. Loops are a powerful tool for data manipulation and achieving various goals in your Python programming.
Using Built-In Functions for Lists in Python
Python provides many built-in functions that make working with lists and performing various operations on them easier. Here's an explanation of some common built-in functions for lists without providing any code:
1. `len()`: This function is used to find the number of elements in the list. For example, if you want to know the number of elements in a list, you can use `len(my_list)`, where `my_list` is the name of the list.
2. `append()`: This function is used to add a new item to the end of the list. For example, you can use `my_list.append(item)` to add `item` to `my_list`.
3. `remove()`: This function is used to remove the first occurrence of a specific value from the list. You can use `my_list.remove(value)` to remove the value `value` from `my_list`.
4. `insert()`: This function is used to insert an item at a specific position within the list. You can use `my_list.insert(index, item)`, where `index` is the location where you want to insert `item`.
5. `pop()`: This function is used to remove and return an item from the end of the list. You can use `my_list.pop()` to remove and retrieve the last item in the list.
6. `index()`: This function is used to search for the first occurrence of a specific value within the list. You can use `my_list.index(value)` to find the position of the value `value` within `my_list`.
7. `count()`: This function is used to count the number of times a specific value appears in the list. You can use `my_list.count(value)` to determine how many times the value `value` appears in `my_list`.
8. `sort()`: This function is used to sort the elements in the list in ascending order. You can use `my_list.sort()` to sort the elements in ascending order.
9. `reverse()`: This function is used to reverse the order of elements in the list. You can use `my_list.reverse()` to reverse the order of elements.
Using these built-in functions can make working with lists in Python easier and more efficient. You can leverage these functions for various purposes such as data searching, transformations, or organizing data according to your programming needs.
How Lists Handle Numbers and Strings
In the Python programming language, lists can easily handle both numbers and strings. Here's how lists deal with these two types of data without providing any code:
1. Storing Numbers and Strings in Lists:
- You can store numbers inside lists without any issues. For example, you can create a list containing integers or floats and use it for mathematical operations and calculations.
- For strings, you can store text strings inside lists with ease. This allows you to organize and manage collections of text, such as user names or items in a shopping list.
2. Accessing Elements:
- Regardless of the data type (numbers or strings) stored in the list, you can access elements using indexes. Lists in Python use an index
Translation of the concept of Lists and Dictionaries in Python and their role in data storage
In the Python programming language, lists can easily and flexibly handle both numbers and text. Here's how lists deal with these two types of data without providing any code:
1. Storing Numbers and Text in Lists:
- You can store numbers inside lists without any problems. For example, you can create a list containing integers or decimals and use it for mathematical operations and calculations.
- For text, you can easily store text strings inside lists. This allows you to organize and manage groups of texts, such as usernames or items in a shopping list.
2. Accessing Elements:
- Regardless of the data type (numbers or text) stored in the list, you can access elements using indices. Lists in Python use indices to access elements, with the index starting at zero.
- For example, if you have a list `my_list`, you can access elements using `my_list[0]` to access the first element in the list.
3. Operations and Arithmetic Operations:
- Using lists, you can perform arithmetic operations like addition, subtraction, multiplication, and division on numbers stored within lists.
- For text, you can perform string operations like concatenation and extracting parts of strings using lists.
4. Multiple Data Types:
- One great feature of lists in Python is their ability to store a mix of different data types. You can put numbers, text, and even other lists within the same list.
- This allows you to create multi-dimensional data structures and organize data flexibly.
5. Loops and Iterative Operations:
- Loops like `for` can be used to iterate over list elements repeatedly. You can easily perform the same operations on all elements.
6. More Operations:
- Python provides a variety of built-in functions and operations that can be used with lists for efficient data searching, manipulation, and organization.
By using these methods and concepts, you can enhance your programming capabilities in Python when dealing with numbers and text within lists effectively and flexibly.
Dictionaries in Python and their role in data storage
Dictionaries are a fundamental data structure in the Python programming language and are very useful for efficiently storing and organizing data. The dictionary in Python is known as a `dictionary` and is an essential part of the built-in data structures.
Now, let's understand the concept of dictionaries and their role in data storage
1. Basic Structure:
- A dictionary in Python is a data structure that stores data in the form of a collection of key-value pairs.
- This means that instead of storing data in an array like lists where it is accessed by indices, data in dictionaries is accessed using unique keys.
2. Keys and Values:
- In dictionaries, each piece of data has a unique key. This key is what you use to access the associated value.
- Values can be of any data type: numbers, strings, lists, other dictionaries, or even custom objects.
3. Primary Use:
- Dictionaries are very useful when you need quick access to data using a specific key.
- For example, dictionaries can be used to store user information in a web application, where you use the user's ID as the key and store other user-related information as values.
4. Working with Dictionaries:
- You can create a new dictionary using curly braces `{}` or by using the built-in `dict()` function.
- You can add key-value pairs to the dictionary and access values using keys easily.
- Additionally, you can modify and delete existing pairs within the dictionary.
5. Use Cases:
- Dictionaries can be used in various scenarios such as data management, storing settings, creating associative arrays, tracking personal information, and much more.
Searching in Dictionaries Using Keys and Accessing Related Values
Searching in dictionaries using keys and accessing related values is an important part of working with dictionaries in Python. You can do this easily using the dictionary's keys. Here is a detailed explanation of how to do that:
1. Using Keys for Search:
- Python dictionaries allow you to search for values using their keys. The key represents the item you want to search for in the dictionary.
2. Accessing Values:
- Once you have searched using the appropriate key, you can access the values associated with that key. Pass the key as an argument to the dictionary using square brackets.
3. Dealing with Non-Existent Keys:
- When you search using a key that doesn't exist in the dictionary, you will typically get a KeyError exception. To avoid this, you can use the `get()` method, which can provide a default value when the key is not found.
4. Checking for Key Existence:
- Before searching for a value using a key, you can also check whether the key exists in the dictionary using the `in` keyword.
5. Using Loops to Iterate Keys and Values:
- You can use loops like the `for` loop to iterate over all keys or values in the dictionary in order.
Using these steps, you can successfully search in dictionaries using keys and access related values in Python. This allows you to organize and retrieve data efficiently according to your needs.
Summary
In conclusion, lists and dictionaries are fundamental parts of the Python language and represent powerful tools for organizing and managing data. Lists allow you to store a variety of elements in a specified order, while dictionaries (such as key-value pairs) provide you with a way to store data in a structured manner.
Whether you are managing arrays of numbers, texts, or even complex data like records, understanding how to use lists and dictionaries and working with them is essential for any Python programmer. These tools allow you to organize data efficiently and perform many important operations such as searching, sorting, updating, adding, and deleting.
By using lists and dictionaries correctly, you can build strong and efficient Python applications based on flexible and user-friendly data structures. Given their importance, it is encouraged to explore more about how to use them and delve into their features to improve your programming skills in Python.
Comment / Reply From
You May Also Like
منشورات شائعة
Newsletter
Subscribe to our mailing list to get the new updates!