Handling Files in Python
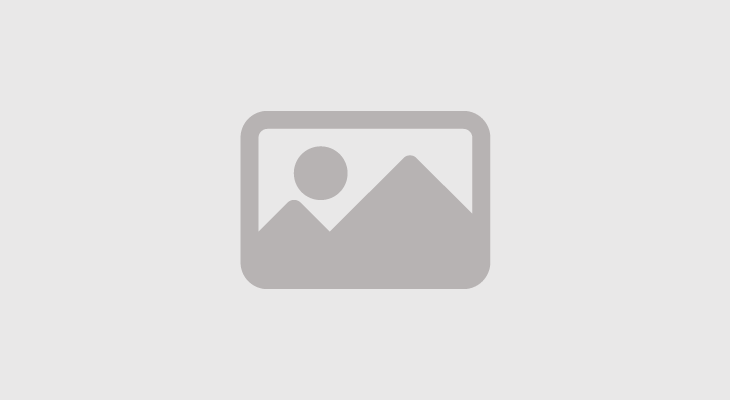
Welcome to the domain of handling files in Python! Efficient file handling is a fundamental aspect of many programming tasks, from reading and writing data to performing complex file manipulations Python provides a comprehensive set of tools and functionalities to seamlessly work with files, making it a versatile language for a wide range of applications
File Operations in Python
In Python, file handling is facilitated through built-in functions and methods that enable the opening, reading, writing, and closing of files This process allows developers to interact with various types of files, from simple text files to binary files and more complex data formats
Reading and Writing
Python's file handling capabilities empower you to read data from files, process it, and write the results back Whether it's parsing a configuration file, analyzing log data, or manipulating large datasets, Python's file handling tools offer flexibility and efficiency
Different File Modes
Files in Python can be opened in different modes such as read mode ('r'), write mode ('w'), append mode ('a'), and binary mode ('b'), among others This flexibility allows you to tailor your file operations to suit specific requirements
Error Handling
Python provides mechanisms for error handling during file operations, ensuring that your programs gracefully manage exceptions that may arise when dealing with files This promotes robust and reliable file processing
Text and Binary Files
Python seamlessly handles both text and binary files Text files are typically used for storing human-readable content, while binary files are employed for a diverse range of applications, including image processing, audio manipulation, and more
As you embark on your exploration of handling files in Python, you'll discover the ease and efficiency with which the language facilitates file-related operations Whether you are working with configuration files, processing data sets, or managing resources, Python's file handling capabilities provide a robust foundation for developing versatile and powerful applications Enjoy harnessing the full potential of Python in handling files as you dive into the world of programming
Handling Files in Python
Handling files in Python is a fundamental and essential process in programming that allows developers to read, write, and manipulate files This aspect of programming is crucial for various applications, whether you are processing text data or performing read and write operations on specialized files such as images or databases Here is an overview of the basic operations for handling files in Python
Opening a File
- The file handling process starts with opening a file using the `open()` function, where you specify the file name and the desired mode (eg, 'r' for reading, 'w' for writing, or 'a' for appending)
- Example
file = open('example.txt', 'r') # Open a file for reading
Reading a File
- You can read the content of the file using functions like `read()` or `readline()` to read one line at a time, or `readlines()` to read all lines into a list
- Example
content = file.read() # Read the entire file content
Writing to a File
- You can write data to the file using the write mode ('w' or 'a' for appending) Functions like `write()` can be used to write data
- Example
with open('output.txt', 'w') as file_out:
file_out.write('Hello, World!')
Working with Records
- When dealing with text files containing separate records (eg, CSV files), you can use functions to read and write records separately
- Example
with open('data.csv', 'r') as csv_file:
for line in csv_file:
# Process each record here
Closing a File
- Always remember to close the file after you're done working with it using the `close()` method to ensure changes are saved, and resources are released
- Example
file.close() # Close the file after finishing the work
Using the `with` Statement
- It is recommended to use the `with` statement when opening files, as it ensures that the file is automatically closed when you are done, helping to avoid forgetting to close the file
- Example
with open('file.txt', 'r') as file:
content = file.read()
# The file is automatically closed here
Handling Errors
- Be sure to handle potential errors during file reading and writing operations using exception handling
By using these fundamentals, you can efficiently handle files in Python and use them in your applications to process and store data effectively
Opening Files in Python The First Step to Dealing with Content
Opening files using Python is the crucial first step towards dealing with file content You can use Python to open text and binary files, read from them, or write to them Here is the detail on how to open files using Python
Using the `open()` Function
The `open()` function in Python is used to open a file You need to provide the file name as one of the arguments, and you can also provide another argument to express the opening mode, such as "read" (for reading), "write" (for writing), or "append" (to add more content to the end of the current file)
Opening in Read Mode
To open a file for reading, you can use the mode `'r'` as an argument for the `open()` function This allows you to read the content only
Opening in Write Mode
To open a file for writing, you can use the mode `'w'` as an argument for the `open()` function If the file already exists, its current content will be deleted, and new content will be written
Opening in Append Mode
To open a file for adding more content to its end, you can use the mode `'a'` as an argument for the `open()` function
Opening in Read and Write Mode
You can use the mode `'r+'` to open the file for both reading and writing
Dealing with Binary Files
You can use the `'b'` argument alongside the opening mode to express binary files (eg, images or executables) For example, `'rb'` for reading a binary file
Storing the Opened File in a Variable
You can store the opened file in a variable for later use in various operations like reading and writing
Closing the File after Usage
It is necessary to close the file after you are done using it using the `close()` function to prevent resource leaks and other issues
By following these steps, you can successfully open files using Python and start reading, writing, and manipulating their content as needed for your project
Reading Files in Python How to Use Reading Functions
Reading files in Python is an important process that allows you to read the content stored in a text or data file Here are guidelines on how to use reading functions without the need for specific code
Open the File for Reading
Before reading, you must open the file you want to read using the `open()` function You can pass the file name and the opening mode `'r'` as an argument to the function This means you are opening the file for reading only
Read the File in the Appropriate Way
Once the file is open, you can use various Python functions to read the content Examples of these functions include
- `read()` Reads the entire content of the file and stores it in a text variable
- `readline()` Reads one line from the file at a time
- `readlines()` Reads all lines in the file and stores them in a list
Process the Read Content
After reading the content from the file, you can process it as needed You can analyze the text, split it into sections, or use it in other operations according to your project's requirements
Close the File After Use
After finishing reading the file and working on it, you should close the file using the `close()` function This helps release resources and prevents potential access issues
Handling Errors
Always keep in mind that reading operations can lead to errors You can use a try-except structure to handle these errors and take appropriate actions when they occur
By following these steps, you can easily use reading functions in Python to read files and process their content as needed Remember always to close the file after use to ensure proper data storage and to avoid security and performance issues
Writing Files in Python Saving Data in Text Files
Writing files in Python involves the process of saving data in text files Here is a detailed explanation of how to do this without the need for specific code
Open the File for Writing
To start writing a text file, you need to open the file using the `open()` function You can pass the file name and the opening mode `'w'` as an argument to the function This means you are opening the file for writing
Write Data
Once you have opened the file for writing, you can use various Python functions to write data to the file Examples of these functions include
- `write()` Writes text or data to the file
- `writelines()` Writes a collection of strings to the file
Close the File After Writing After finishing writing data to the file, you should close it using the `close()` function This helps release resources and applies the written data
Handling Errors
You should be cautious and handle any errors that may occur during the writing process You can use a try-except structure to manage these errors and take appropriate actions
Checking File Existence
You can use the `ospathexists()` function to check whether the file exists before attempting to open it for writing This can help avoid errors resulting from attempting to write to a non-existent file
Using Append Mode
If you want to add more data to an existing file without erasing the previous content, you can use the `'a'` mode instead of `'w'` when opening the file
By following these steps, you can easily write files in Python and save data in text files as needed Always make sure to close the file after writing to ensure proper data preservation and to avoid security and performance issue
Dealing with CSV Files Using Python Extracting Organized Data
Handling CSV files using Python means extracting organized data from CSV files CSV files are text files used to store data in tabular form with rows and columns, where values are separated by a specified delimiter, such as a comma or a semicolon
Here's a detailed explanation of how to extract data from CSV files using Python
Understand the CSV File Structure
Before you begin extracting data from a CSV file, you need to understand the file's structure This means identifying the rows and columns present in the file and how the data is organized
Use the CSV Library
In Python, you can use the built-in CSV library to work with CSV files You can import this library using `import csv`, and then use the functions and objects provided by this library to read CSV files
Open the CSV File
You should use the `open()` function to open the CSV file You can pass the file name and the appropriate mode (read or write) to the function
Prepare a CSV Reader
After opening the file, you should prepare a CSV reader using the `csvreader()` function This reader will help you read the data in an organized manner
Extract Data
You can now use the CSV reader to read data from the file You can access rows in the file and extract data from columns by iterating through the rows
Data Processing
Once you've extracted the data, you can process it as needed You can convert values to different data types, clean the data, or perform any other operations required
Close the File
After extracting and processing the data, you should close the CSV file using the `close()` function to release resources
Handling Errors
You should be cautious and handle any errors that may occur during the data extraction process You can use a try-except structure to manage these errors and take appropriate actions
With these steps, you can extract organized data from CSV files using Python without the need for writing specific code This allows you to effectively use the data for analysis or in your projects
Searching and Replacing in Text Files Using Python Modifying Content
Searching and replacing in text files using Python means modifying the content within a text file by finding a specific text and replacing it with another text This process can be used to update data, clean text, or make any other changes to the file
Here's an explanation of how to perform searching and replacing in text files using Python without the need for specific code
Understand the File Structure
Before starting the search and replace process, you should understand the structure of the file and the text you want to change You need to know the text you're searching for and the text you want to replace it with
Select the Target File
Choose the text file where you want to perform the search and replace operation The file can be local on your computer or online
Use a Text Editor
To manually search and replace within the file, you can use any text editor that displays the file's content clearly Look for the "Find and Replace" function in the text editor to perform this operation
Search for Text
Enter the text you want to search for in the search box You can use options like case-sensitive search or finding similar words to search for the text accurately
Replace Text
After finding the text you want to replace, enter the new text you want to replace it with Then, click the "Replace" or "Replace All" button if you want to replace all instances of the matching text
Save Changes
Once you've finished the replace operation, save the file You can use the "Save" or "Save As" option if you want to keep a copy of the original file
Verify Changes
Reopen the file to check that the changes have been successfully implemented Ensure that the new text has been replaced correctly
These are the basic steps for searching and replacing in text files using any text editor You can repeat this process for many files, and with Python, you can also write programs that perform these types of operations automatically without manual intervention
Closing Files in Python Ensuring Resource Release
Closing files in Python is an important step to ensure the proper release of resources and manage files correctly When you open a file in Python using a function like `open()`, it's crucial to always make sure to close the file after you're done using it Here's a detailed explanation of how to close files in Python and its importance
Open the File
When working with a file, you start by opening it using the `open()` function in Python This function is used to open the file for reading, writing, or appending
Work on the File
Once the file is open, you can read its contents, write new data to it, or perform any other operations as needed
Close the File
After you finish working on the file, you should close it using the `close()` function in Python This step is important for several reasons
- Resource Release
Closing the file releases the resources that were used to access the file, helping conserve system resources
- Data Preservation
When you close the file properly, any changes made to it are saved and applied If you don't close the file properly, you may lose changes that haven't been preserved
- Secure File Access
Closing the file makes it unavailable for reading or writing by other programs, preventing interference between read and write operations
Use the `with` Structure
Instead of using `close()` separately, it's recommended to use the `with` structure in Python for automatically opening and closing files This ensures that the file is closed automatically when you're done working with it within the `with` block
In summary, closing files in Python is essential to avoid resource leaks, ensure proper data preservation, and allow secure file access If you fail to close files properly, you may encounter runtime issues and potential data loss
Dealing with Large Files Efficiently in Python
Efficiently handling large files in Python involves a set of ideas and techniques aimed at improving the performance of reading and writing operations for large files Here are some tips on how to efficiently handle large files
Use Streaming Reads
When reading a large file, use streaming reads with a `for` loop to read the file iteratively instead of reading the entire file into memory This reduces memory consumption and allows for processing large files
Use Chunking
You can divide a large file into smaller parts called "chunks" and read them sequentially This can be useful if you want to process data chunk by chunk rather than reading the entire file
Utilize External Libraries
In some cases, you can use external libraries like "Pandas" to efficiently handle large files These libraries come with tools for reading and processing large files easily
Process Data in a Streaming Manner
When reading or writing, try to process the data in a streaming manner rather than storing it all in memory This helps reduce memory consumption and speeds up processing
Use Binary Read/Write Mode
For reading or writing very large binary files, such as image or video files, consider using binary read/write mode to improve performance and efficiency
Clean Up Large Files
Before processing a large file, you can review the file and filter out unnecessary data if possible This reduces the file size and makes it more manageable
Employ Multi-threading/Multi-processing
When dealing with extremely large files, you can use multi-processing techniques to increase performance You can divide the file and process different parts of it concurrently
Avoid Repeated Reads
If you need to access the same file multiple times, read it once and store the data in data structures like dictionaries or lists to avoid repeated reads
Monitor Resource Usage
Track resource consumption while dealing with large files using resource monitoring tools like "psutil" to ensure that your application doesn't run into performance or memory issues
Use Data Compression
In some cases, data compression techniques can be used to reduce the size of large files before handling them
When dealing with large files in Python, it's important to carefully plan for maximum performance efficiency, avoid excessive memory consumption, and optimize processing speed
Backing up and restoring files using Python
Backing up and restoring files are crucial operations for preserving data and preventing loss or corruption Here's an explanation of how to perform these operations using Python
Backing up files using Python
Select the target files and directories Before starting the backup process, identify the files and directories you want to back up You can use Python libraries like `os` to access files and directories on the system
Choose a destination path for the backup Decide where you want to store the backup This could be a dedicated folder or an external storage device
Create the backup file Using a Python library like `shutil`, use the `copy()` or `copytree()` function to copy the selected files and directories to the chosen backup destination
Store additional information If you have any additional information about the backup, such as its date or description, you may want to record this information in a separate file or database
Verify the success of the backup After completing the backup process, verify that the files were successfully copied and are present in the backup location
Restoring files using Python
Select the target restore path Before starting to restore files, specify the location to which you will restore them This should be a secure location designated for restored files
Choose the files to restore Select the files you want to restore from the backup These could be all files or only those that were deleted or lost
Use a Python library for restoration Using a Python library like `shutil`, use the `copy()` function to copy the restored files to the chosen restore destination
Verify the success of the restoration After completing the restoration process, verify that the files were successfully restored to the designated location
Restore additional information (optional) If you have additional information about the restored files that needs to be reinstated, such as permissions or descriptions, you may need to configure this information accordingly
Check and test restored files Before fully relying on the restored files, open and verify them to ensure they are intact and functioning correctly
Always remember the importance of regularly performing backup operations and verifying their success to ensure the safety of critical data and files
Tips and tricks for improving file handling performance in Python
Improving file handling performance in Python depends on the strategies and techniques you employ Here are some tips and tricks that can help you enhance file handling performance
Use Context Managers
Use context managers, such as `with`, to ensure that files are automatically closed after use This reduces the likelihood of resource leaks and improves performance
Utilize Buffering
When dealing with large files, consider using buffering to reduce the number of input and output operations, which can significantly enhance performance
Leverage the os Library for File Operations
The os library in Python provides efficient functions for file and directory operations, contributing to improved performance and efficiency
Avoid Repeatedly Opening and Closing Files
If you need to read or write multiple files, open them once and reuse them for sequential operations instead of repeatedly opening and closing files
Use Text Mode Instead of Binary Mode
Unless there is a genuine need to work with binary data, use text mode (with "r," "w," "a," "t," etc) for file reading and writing
Utilize Embedded Lists
When reading data from files like CSV, JSON, or XML, use embedded lists to organize and store hierarchical data
Optimize Search Operations
If you're searching for specific data within large files, employ efficient search techniques, such as indexing or associative lists
Avoid Over-Encrypting Files
If there's no real need to encrypt files, skip this step as it adds extra overhead and may reduce performance
Use Specialized Libraries When dealing with specific file types like CSV or Excel, you may find specialized libraries like Pandas or openpyxl that offer optimized tools for your task
Use Advanced Reading and Writing Libraries
For handling multi-dimensional data more efficiently, you may need to use external libraries like NumPy
Maintain File Security
Before applying any changes to files, ensure that you have securely copied them and maintain backups to safeguard data
Measure and Analyze Performance
Employ performance analysis tools like cProfile to measure and optimize file handling performance and identify any performance bottlenecks
Improving file handling performance in Python relies on a good understanding of project requirements and the use of appropriate tools and techniques to achieve optimal performance Avoid unnecessary operations and leverage Python libraries and efficient programming practices to make the most of your file handling operations
Summary
In conclusion, file handling in Python is a fundamental and necessary process that requires a good grasp of fundamentals and advanced concepts "File handling in Python" refers to the ability to efficiently and securely open, read, write, analyze, and process files Python offers many tools and libraries that facilitate these operations
Comment / Reply From
You May Also Like
منشورات شائعة
Newsletter
Subscribe to our mailing list to get the new updates!